|
Depolarizer * | clone () const |
| Clone operator.
|
|
std::string | get_name () const |
| Return the name of the class.
|
|
| Evaluable () |
| Default constructor.
|
|
| Evaluable (const Evaluable ©) |
| Copy constructor.
|
|
Evaluable & | operator= (const Evaluable ©) |
| Assignment operator.
|
|
Matrix< 4, 4, double > | evaluate (std::vector< Matrix< 4, 4, double > > *grad=0) const |
| Return the Jones matrix and its gradient.
|
|
EstimateTraits< Matrix< 4, 4, double > >::type | estimate () const |
| Return the Jones Estimate matrix.
|
|
| Function () |
| Default constructor.
|
|
| Function (const Function &model) |
| Copy constructor.
|
|
Function & | operator= (const Function &model) |
| Assignment operator.
|
|
virtual | ~Function () |
| Virtual destructor.
|
|
virtual void | copy (const Function *model) |
| Does the work for operator =.
|
|
virtual TextInterface::Parser * | get_interface () |
| Return a text interface that can be used to access this instance.
|
|
virtual void | parse (const std::string &text) |
| Parses the values of model parameters and fit flags from a string.
|
|
virtual void | print (std::string &text) const |
| Prints the values of model parameters and fit flags to a string.
|
|
virtual std::string | get_description () const |
| Return the description of the class.
|
|
unsigned | get_nparam () const |
| Return the number of parameters.
|
|
std::string | get_param_name (unsigned index) const |
| Return the name of the specified parameter.
|
|
std::string | get_param_description (unsigned index) const |
| Return the description of the specified parameter.
|
|
double | get_param (unsigned index) const |
| Return the value of the specified parameter.
|
|
void | set_param (unsigned index, double value) |
| Set the value of the specified parameter.
|
|
double | get_variance (unsigned index) const |
| Return the variance of the specified parameter.
|
|
void | set_variance (unsigned index, double value) |
| Set the variance of the specified parameter.
|
|
bool | get_infit (unsigned index) const |
| Return true if parameter at index is to be fitted.
|
|
void | set_infit (unsigned index, bool flag) |
| Set flag for parameter at index to be fitted.
|
|
void | set_argument (unsigned dimension, Argument *axis) |
| Set the independent variable of the specified dimension.
|
|
Estimate< double > | get_Estimate (unsigned index) const |
| Return an Estimate of the specified parameter.
|
|
void | set_Estimate (unsigned index, const Estimate< double > ¶m) |
| Set the Estimate of the specified parameter.
|
|
void | set_verbose (bool) |
| Set the verbosity of this instance.
|
|
bool | get_verbose () const |
| Get the verbosity of this instance.
|
|
void | set_evaluation_changed (bool _changed=true) |
| Set true if the Function evaluation has changed.
|
|
bool | get_evaluation_changed () const |
| Return true if the Function evaluation has changed.
|
|
const ParameterPolicy * | get_parameter_policy () const |
| Provide access to the parameter_policy attribute.
|
|
bool | has_parameter_policy () const |
| Some wrappers may not have a parameter policy.
|
|
virtual void | print_parameters (std::string &text, const std::string &separator) const |
| Prints the values of model parameters and fit flags to a string.
|
|
| Able (const Able &) |
|
Able & | operator= (const Able &) |
|
unsigned | get_reference_count () const |
|
| HeapTracked (const HeapTracked &) |
|
HeapTracked & | operator= (const HeapTracked &) |
|
bool | __is_on_heap () const |
|
|
void | calculate (Matrix< 4, 4, double > &result, std::vector< Matrix< 4, 4, double > > *) |
| Calculate the Mueller matrix and its gradient. More...
|
|
void | calculate (Evaluable *eval, Matrix< 4, 4, double > &result, std::vector< Matrix< 4, 4, double > > *grad) |
| Use the calculate method of another Evaluable instance.
|
|
void | copy_evaluation_policy (const Evaluable *other) |
|
void | copy_evaluation_changed (const Function &model) |
| Copy the evaluation changed state of another model instance.
|
|
void | copy_parameter_policy (const Function *) |
| Copy the parameter policy of another instance.
|
|
void | set_parameter_policy (ParameterPolicy *policy) |
| Set the parameter policy.
|
|
Handle * | __reference (bool active) const |
|
void | __dereference (bool auto_delete=true) const |
|
|
typedef Matrix< 4, 4, double > | Result |
| The return type of the evaluate method.
|
|
enum | Attribute { ParameterCount,
Evaluation
} |
| Function attributes that require the attention of Composite models. More...
|
|
template<class Model > |
static Model * | load (const std::string &filename) |
| Construct a new Model instance from a file.
|
|
static Function * | load_Function (const std::string &filename) |
| Construct a new Function instance from a file.
|
|
static Function * | factory (const std::string &text) |
| Construct a new Function instance from a string. More...
|
|
static size_t | get_instance_count () |
|
static void * | operator new (size_t size, void *ptr=0) |
|
static void | operator delete (void *location, void *ptr) |
|
static void | operator delete (void *location) |
|
static size_t | get_heap_queue_size () |
|
Callback< Attribute > | changed |
| Callback executed when a Function Attribute has been changed.
|
|
static const char * | Name = "Real4" |
| The name of the class. More...
|
|
static const char * | Name |
| The name of the class.
|
|
static bool | verbose = false |
| Verbosity flag.
|
|
static bool | very_verbose = false |
| Low-level verbosity flag.
|
|
static bool | check_zero = false |
| When set, some Functions will throw an Error if they evaluate to zero.
|
|
static bool | check_variance = false |
| When set, some Functions will throw an Error if input variance <= 0.
|
|
static bool | cache_results = false |
| When set, use the Cached evaluation policy and callbacks.
|
|
Reference::To< EvaluationPolicy< Matrix< 4, 4, double > > > | evaluation_policy |
| The policy for managing function evaluation.
|
|
Reference::To< ParameterPolicy > | parameter_policy |
| The policy for managing function parameters.
|
|
Reference::To< ArgumentPolicy > | argument_policy |
| The policy for managing function arguments.
|
|
bool | set_parameter_policy_context |
| Set the parameter policy context.
|
|
bool | this_verbose |
| The verbosity of this instance.
|
|
Represents a pure depolarizer transformation.
The pure depolarizer is parameterized as described in
Lu, S.H. & Chipman, R.A., 1996, J. Opt. Soc. Am. A, 13, 1106
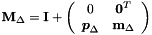
where
is the
identity matrix,
is the 3-vector that describes the polarizance, and
is the
symmetric depolarizer matrix.